Discover techniques for securely storing and handling sensitive data to prevent data breaches and maintain data integrity.
OWASP Best Practices for NextJS (3)
Table of Contents
Secure Data Storage in Next.js
Proper data storage is fundamental for any web application, and Next.js is no different.
This popular React framework facilitates building scalable and performant applications.
However, it’s crucial to understand the potential security risks associated with storing sensitive data in Next.js applications.
This guide explores OWASP’s best practices for secure data storage in Next.js, covering:
- Data Encryption
- Data Validation
- Data Minimization
Data Encryption
Data encryption transforms data into a format that’s unreadable for unauthorized users. This is critical for safeguarding sensitive data like passwords, credit card numbers, and personal information.
Next.js offers built-in methods for data encryption. Here are two options:
next-iron-session
package: This provides a secure and user-friendly way to store encrypted data in cookies.next-auth
package: This offers a comprehensive authentication and authorization solution that includes data encryption.
Data Validation
Data validation ensures data accuracy and completeness before storage. This is vital to prevent errors and malicious attacks.
Next.js provides built-in options for data validation, including:
yup
package: This offers a powerful and easy-to-use approach to data validation.validator
package: This provides a simple and lightweight solution for data validation.
Data Minimization
Data minimization involves collecting and storing only the data that’s absolutely necessary. This reduces the risk of data breaches and unauthorized access.
Next.js offers built-in methods for data minimization, such as:
SWR package: This enables fetching data from a server-side API and caching it on the client-side. This helps reduce the amount of data stored on the client.
Data Validation and Sanitization for Next.js Apps
Protecting Your Next.js Apps: Data Validation and Sanitization
In web development, data validation and sanitization are critical for securing user input and preventing malicious attacks. These techniques ensure the data you receive from users adheres to your expectations and is free from harmful characters or code.
By implementing robust data validation and sanitization practices, you can significantly strengthen the security of your Next.js applications.
Understanding the Concepts:
-
Data Validation: This verifies that user input conforms to predefined rules and constraints. It checks for expected data types, ranges, and formats.
For example, ensuring a user’s name only contains letters or that a date field follows a specific format (e.g., YYYY-MM-DD). -
Data Sanitization: This involves removing or modifying malicious or unwanted characters and code from the input. It safeguards your application from attacks like cross-site scripting (XSS) and SQL injection.
Common techniques include encoding special characters, trimming whitespace, and removing potentially dangerous tags and attributes.
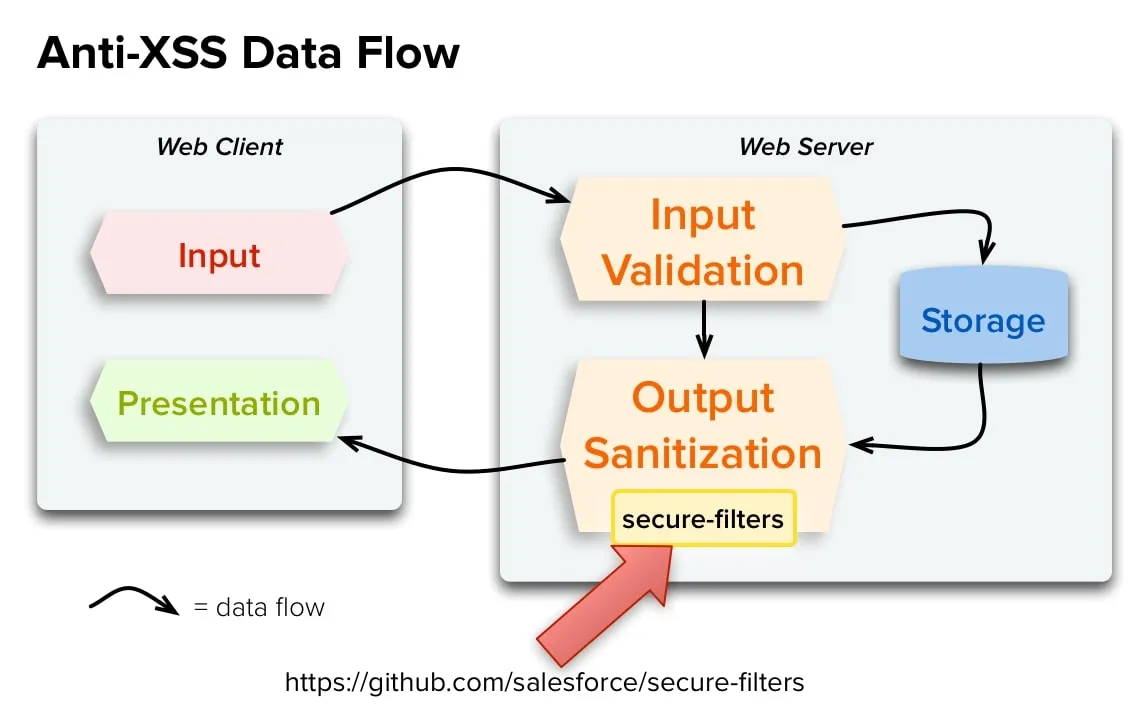
Best Practices for Next.js:
- Utilize Existing Libraries: Leverage libraries like
validator.js
oryup
for comprehensive input validation. They offer pre-defined rules and sanitization functions, streamlining the process. - Multi-Layered Protection: Perform data validation on both the server-side and client-side. This prevents malicious input from reaching your server and compromising your application.
- Sanitize Before Processing: Always sanitize user input before storing or processing it. Encode special characters, remove tags, and trim whitespace to prevent malicious code execution.
- Regular Expressions for Complex Validation: Regular expressions are powerful tools for validating specific patterns in user input. They allow defining complex rules to ensure input matches the expected format.
- OWASP Guidelines: Refer to the OWASP (Open Web Application Security Project) guidelines for data validation and sanitization best practices. These guidelines provide a comprehensive set of recommendations to secure your applications against common attacks.